Fund user wallets
Funding methods
In order for users to fully participate in a game economy, such as by purchasing items, paying for transactions or trading with other users, they need to have the game's native currency, or other game-accepted currencies, in the wallets they have connected to the game. We have developed the Commerce Widget as an easy to use tool designed to facilitate this process.
Flow | Use case |
---|---|
Bridge | The BRIDGE flow is ideal for users who have funds on L1 (e.g. Ethereum) and are looking to move some of the funds to zkEVM. 💡Example An item is sold on zkEVM for 0.2 USDC and I have 3 USDC in my L1 wallet that I'd love to use for purchasing the item. I can use the Checkout widget to bridge my L1 USDC tokens to L2 and complete the purchase. |
Swap | The SWAP flow is ideal for users who have funds on zkEVM and are looking to swap tokens (e.g. USDC for IMX). 💡Example An item is sold on zkEVM for 0.2 USDC and I already have 4 ETH in my zkEVM wallet that I'd love to use for purchasing the item. I can use the Checkout widget to swap my ETH tokens for USDC tokens and complete the purchase. |
On‑ramp | The ONRAMP flow is idea for users who want to funds their zkEVM wallets using fiat (e.g. EUR). 💡Example An item is sold on zkEVM for 0.2 USDC and I do not have any funds on zkEVM on L1 (e.g. Ethereum). I can use the Checkout widget to purchase USDC tokens using my Credit Card/Apple Pay/Google Pay and complete the purchase. |
Setup
The Commerce Widget is designed to be used client-side only and can be installed via CDN or package manager.
- npm module
- JavaScript via CDN
Prerequisites
Node Version 20 or later
To install nvm
follow these instructions. Once installed, run:
nvm install --lts
- (Optional) To enable Code Splitting (importing only the SDK modules you need) there are additional prerequisites.
Install the Immutable SDK
Run the following command in your project root directory.
- npm
- yarn
npm install -D @imtbl/sdk
# if necessary, install dependencies
npm install -D typescript ts-node
yarn add --dev @imtbl/sdk
# if necessary, install dependencies
yarn add --dev typescript ts-node
The Immutable SDK is still in early development. If experiencing complications, use the following commands to ensure the most recent release of the SDK is correctly installed:
- npm
- yarn
rm -Rf node_modules
npm cache clean --force
npm i
rm -Rf node_modules
yarn cache clean
yarn install
To add the SDK to an application using the CDN the following script can be placed inside the head tag.
<script src="https://cdn.jsdelivr.net/npm/@imtbl/sdk/dist/browser/checkout/sdk.js"></script>
Bridge
Bridges facilitate communication between blockchains through the transfer of information and assets. The sample code code below gives a starting point for integrating the widget into your application.
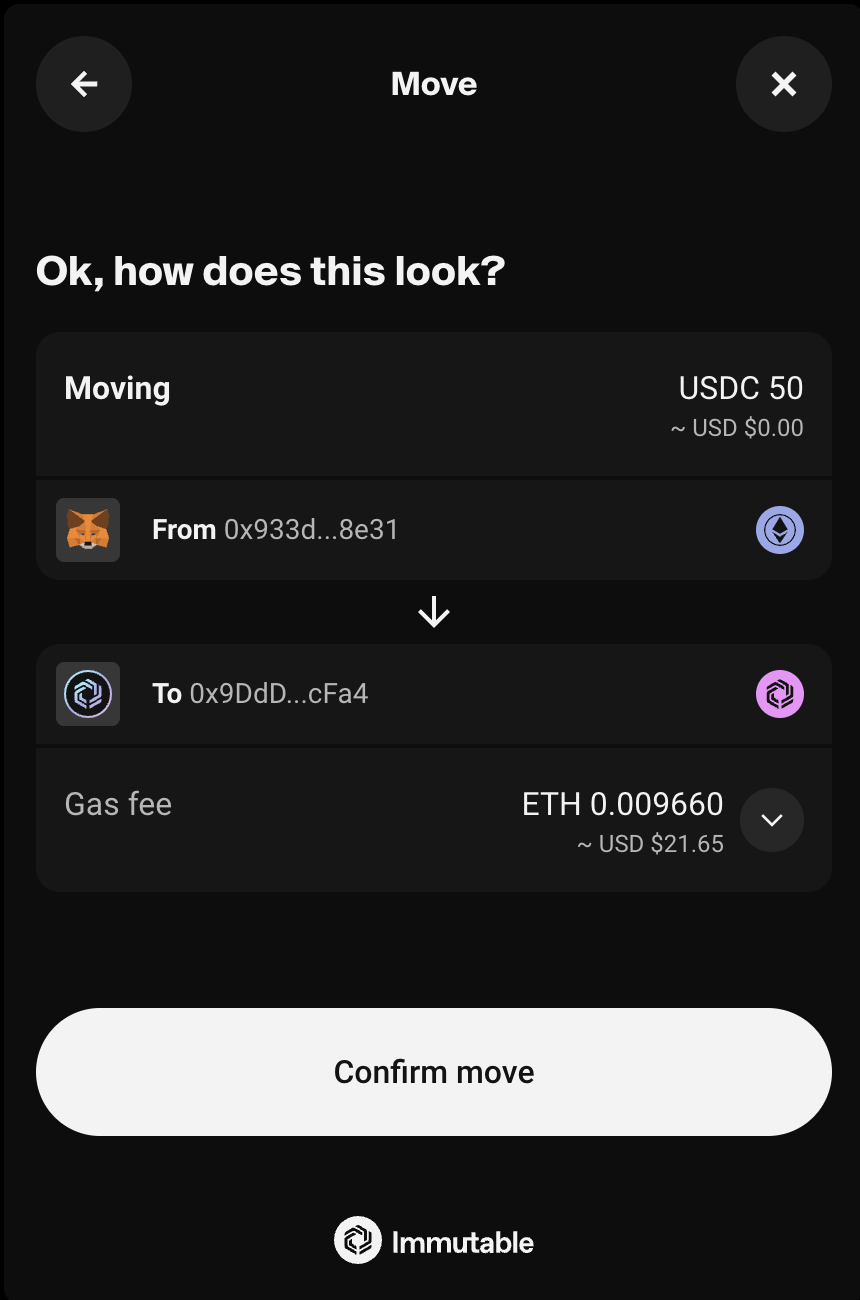
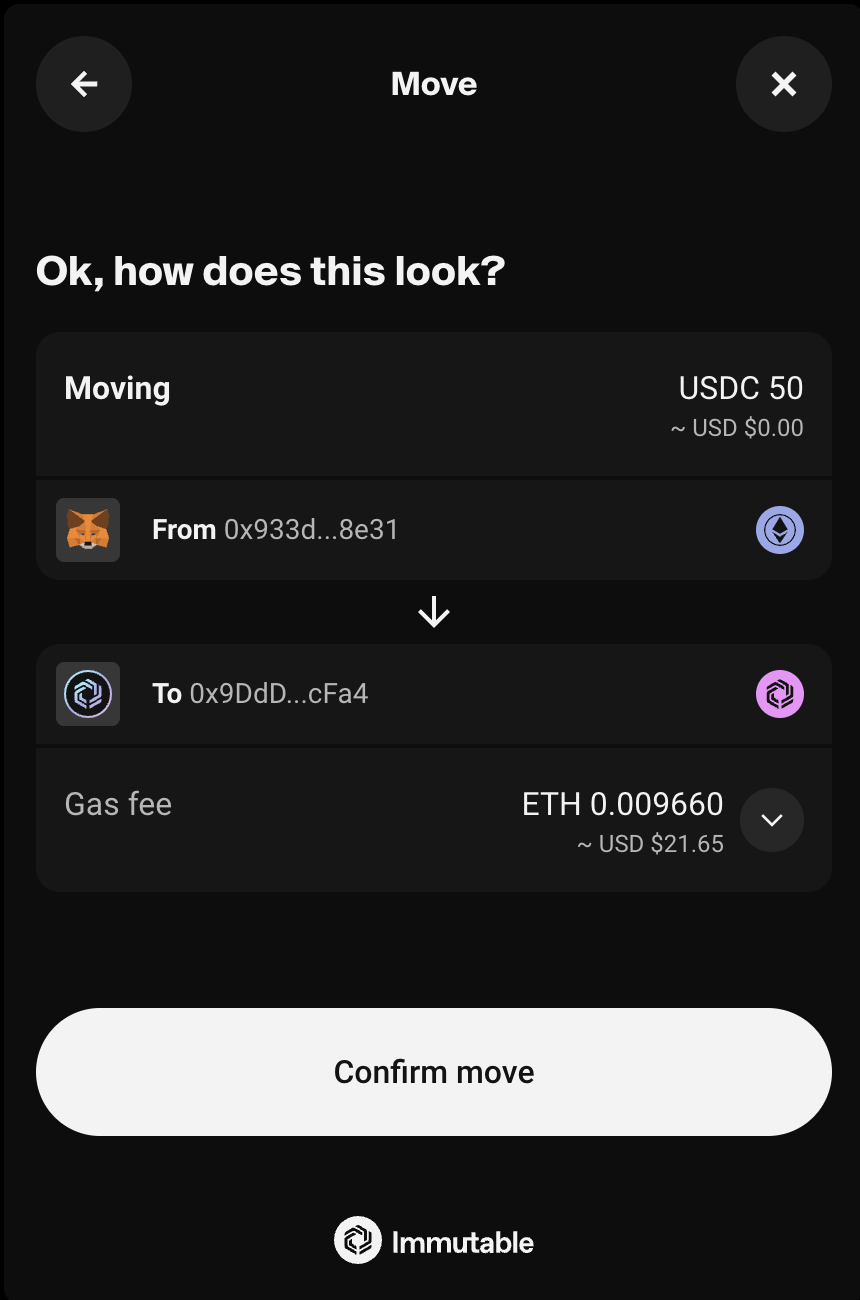
- React
- JavaScript
import { useEffect } from 'react';
import { checkout } from '@imtbl/sdk';
// Create a Checkout SDK instance
const checkoutSDK = new checkout.Checkout();
export function App() {
// Initialise the Commerce Widget
useEffect(() => {
(async () => {
// Create a factory
const factory = await checkoutSDK.widgets({
config: { theme: checkout.WidgetTheme.DARK, language: 'en' },
});
// Create a widget
const widget = factory.create(checkout.WidgetType.IMMUTABLE_COMMERCE);
// Mount a bridge flow, optionally pass any BridgeWidgetParams
widget.mount('mount-point', {
flow: checkout.CommerceFlowType.BRIDGE,
});
})();
}, []);
return <div id="mount-point" />;
}
<html>
<head>
<!-- Load the SDK from jsdelivr -->
<script src="https://cdn.jsdelivr.net/npm/@imtbl/sdk/dist/browser/checkout/sdk.js"></script>
</head>
<body>
<div id="mount-point"></div>
<script>
// Initialize Checkout SDK
var checkout;
(async function () {
checkout = new ImmutableCheckout.Checkout();
const factory = await checkout.widgets({
config: { theme: ImmutableCheckout.WidgetTheme.DARK },
});
const widget = factory.create(
ImmutableCheckout.WidgetType.IMMUTABLE_COMMERCE
);
widget.mount('mount-point', {
flow: ImmutableCheckout.CommerceFlowType.BRIDGE,
});
})();
</script>
</body>
</html>
To learn more about this widget explore the Bridge tokens documentation.
Swap
A token swap refers to exchanging one cryptocurrency token directly for another without using fiat money (e.g. USD). The sample code code below gives a starting point for integrating the widget into your application.
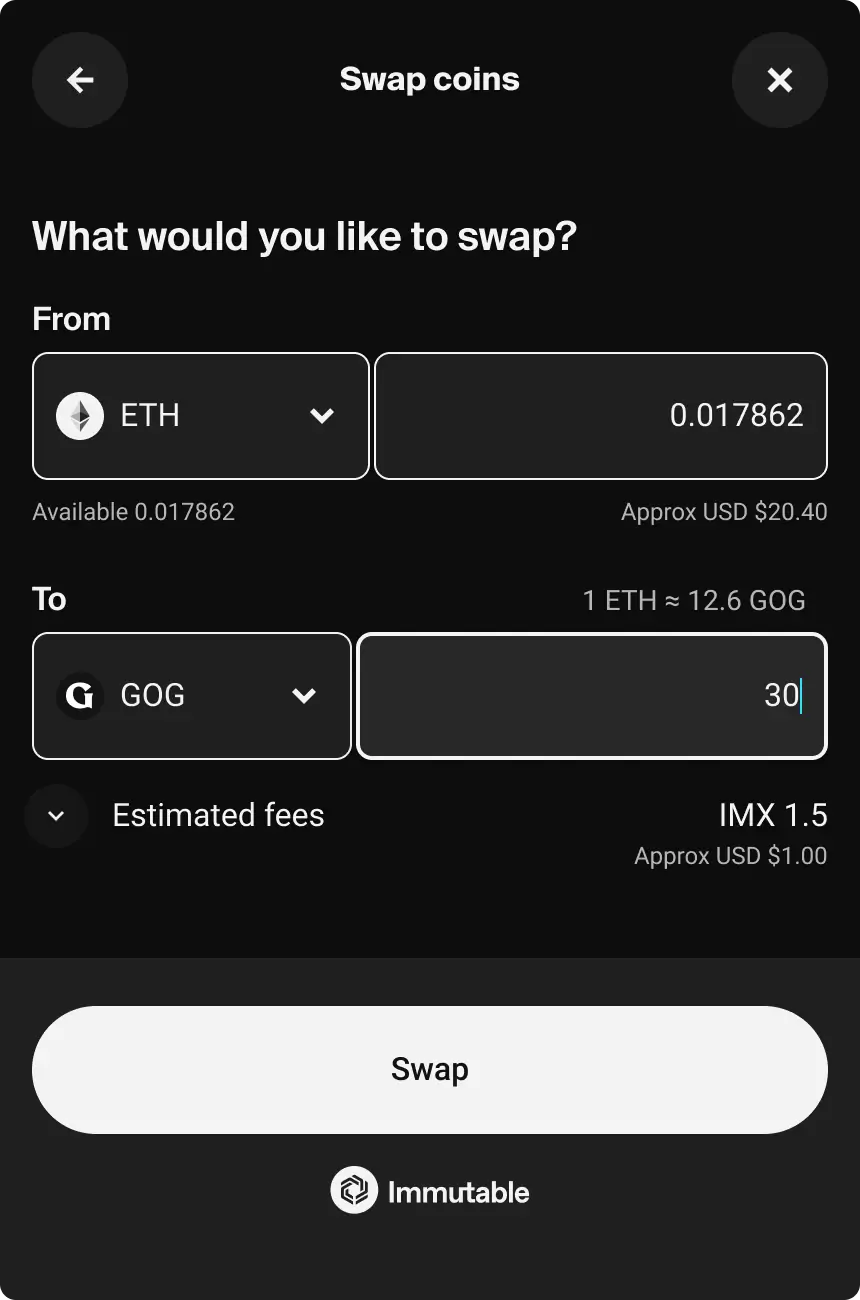
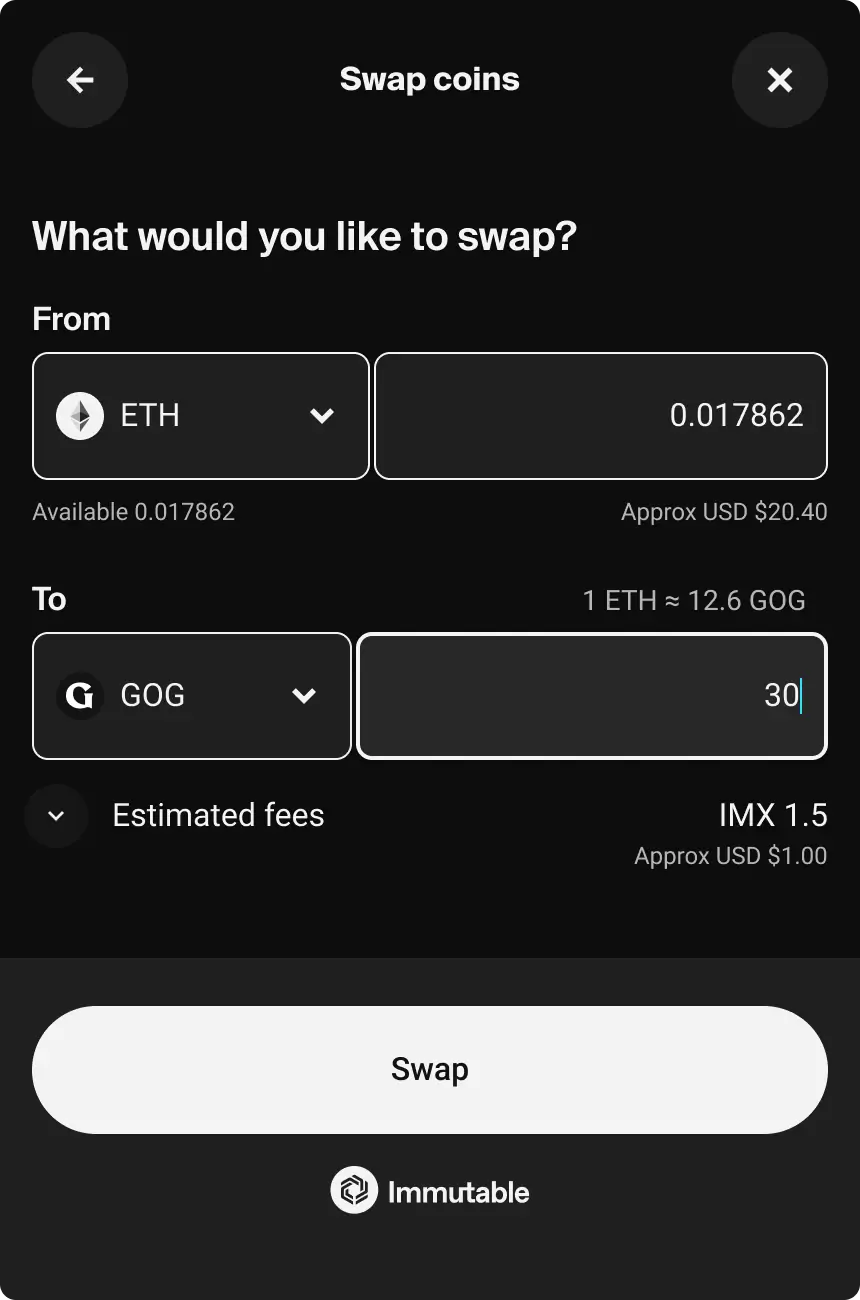
- React
- JavaScript
import { useEffect } from 'react';
import { checkout } from '@imtbl/sdk';
// Create a Checkout SDK instance
const checkoutSDK = new checkout.Checkout();
export function App() {
// Initialise the Commerce Widget
useEffect(() => {
(async () => {
// Create a factory
const factory = await checkoutSDK.widgets({
config: { theme: checkout.WidgetTheme.DARK, language: 'en' },
});
// Create a widget
const widget = factory.create(checkout.WidgetType.IMMUTABLE_COMMERCE);
// Mount a SWAP flow, optionally pass any SwapWidgetParams
widget.mount('mount-point', {
flow: checkout.CommerceFlowType.SWAP,
});
})();
}, []);
return <div id="mount-point" />;
}
<html>
<head>
<!-- Load the SDK from jsdelivr -->
<script src="https://cdn.jsdelivr.net/npm/@imtbl/sdk/dist/browser/checkout/sdk.js"></script>
</head>
<body>
<div id="mount-point"></div>
<script>
// Initialize Checkout SDK
var checkout;
(async function () {
checkout = new ImmutableCheckout.Checkout();
const factory = await checkout.widgets({
config: { theme: ImmutableCheckout.WidgetTheme.DARK },
});
const widget = factory.create(
ImmutableCheckout.WidgetType.IMMUTABLE_COMMERCE
);
widget.mount('mount-point', {
flow: ImmutableCheckout.CommerceFlowType.SWAP,
});
})();
</script>
</body>
</html>
To learn more about this widget explore the Swap Tokens documentation.
On-ramp
On-ramping tokens refers to exchanging fiat currency (e.g. USD) directly to buy cryptocurrency. The sample code code below gives a starting point for integrating the ONRAMP flow into your application.
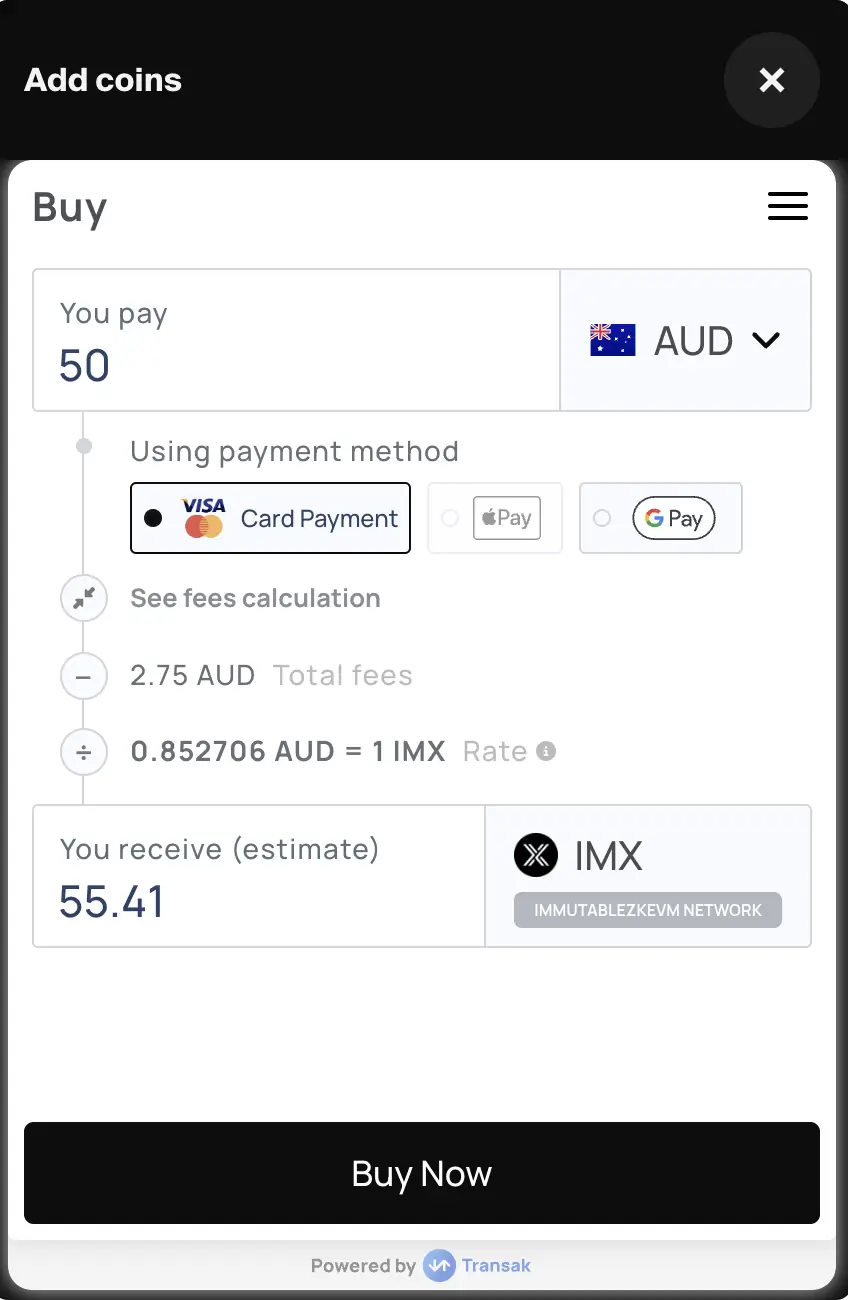
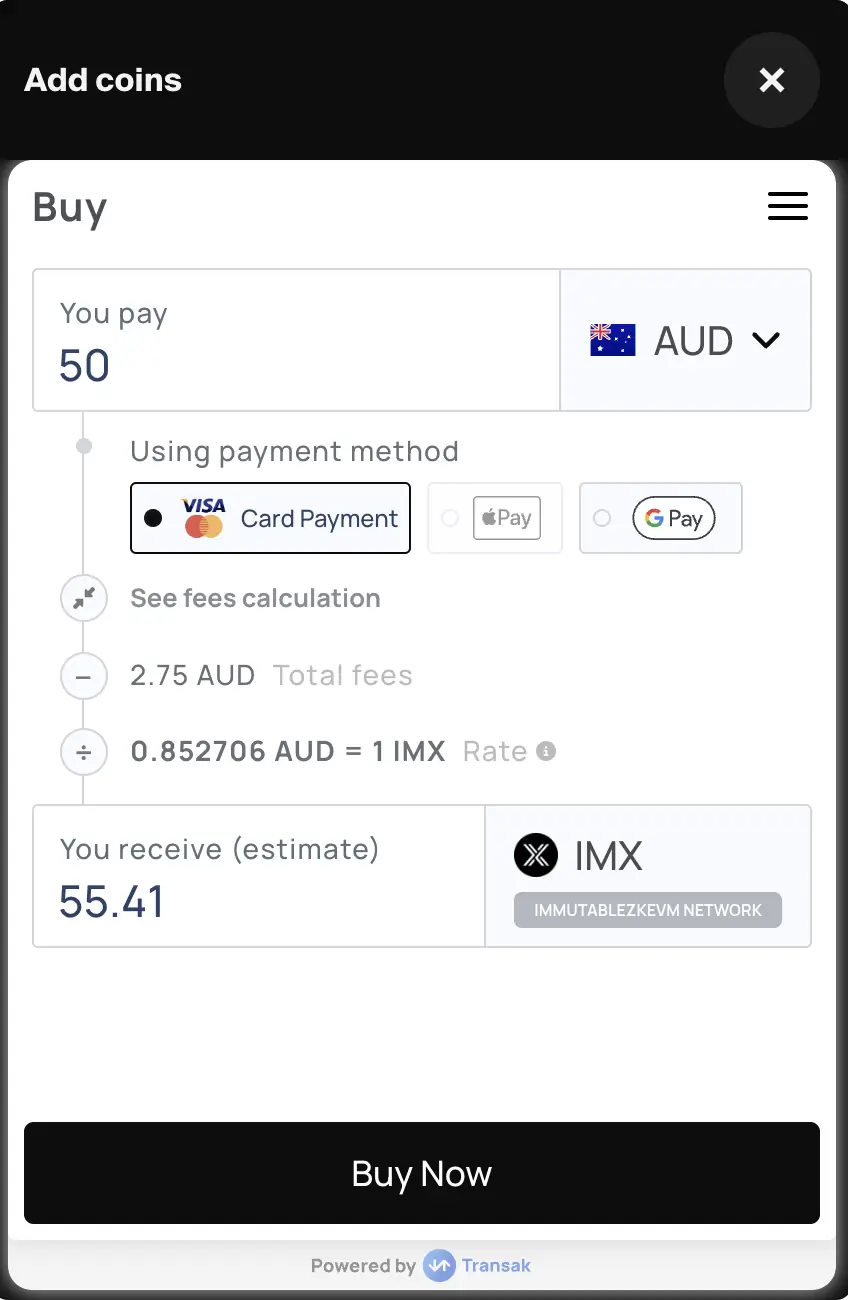
- React
- JavaScript
import { useEffect } from 'react';
import { checkout } from '@imtbl/sdk';
// Create a Checkout SDK instance
const checkoutSDK = new checkout.Checkout();
export function App() {
// Initialise the Commerce Widget
useEffect(() => {
(async () => {
// Create a factory
const factory = await checkoutSDK.widgets({
config: { theme: checkout.WidgetTheme.DARK, language: 'en' },
});
// Create a widget
const widget = factory.create(checkout.WidgetType.IMMUTABLE_COMMERCE);
// Mount an onramp flow, optionally pass any OnrampWidgetParams
widget.mount('mount-point', {
flow: checkout.CommerceFlowType.ONRAMP,
});
})();
}, []);
return <div id="mount-point" />;
}
<html>
<head>
<!-- Load the SDK from jsdelivr -->
<script src="https://cdn.jsdelivr.net/npm/@imtbl/sdk/dist/browser/checkout/sdk.js"></script>
</head>
<body>
<div id="mount-point"></div>
<script>
// Initialize Checkout SDK
var checkout;
(async function () {
checkout = new ImmutableCheckout.Checkout();
const factory = await checkout.widgets({
config: { theme: ImmutableCheckout.WidgetTheme.DARK },
});
const widget = factory.create(
ImmutableCheckout.WidgetType.IMMUTABLE_COMMERCE
);
widget.mount('mount-point', {
flow: ImmutableCheckout.CommerceFlowType.ONRAMP,
});
})();
</script>
</body>
</html>
To learn more about this widget explore the On-ramp tokens documentation.